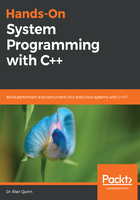
Scope
One major difference between C and C++ is how the construction and destruction of an object is handled. Let's take the following example:
#include <iostream>
struct mystruct {
int data1{42};
int data2{42};
};
int main(void)
{
mystruct s;
std::cout << s.data1 << '\n';
}
// > g++ scratchpad.cpp; ./a.out
// 42
Unlike in C, in C++ we are able to use the {} operator to define how we would like the data values of the structure to be initialized. This is possible because, in C++, objects (both structures and classes) contain constructors and destructors that define how the object is initialized on construction and destroyed on destruction.
When system programming, this scheme will be used extensively, and the idea of the construction and destruction of objects will be leveraged throughout this book when handling system resources. Specifically, a scope will be leveraged to define the lifetime of an object, and thus the system resource that the object owns, using a concept called Resource Acquisition is Initialization (RAII).