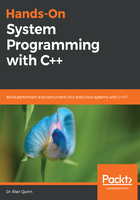
Boolean
The standard C language doesn't define a Boolean type natively. C++, however, does, and is defined using the bool keyword. When writing in C, a Boolean can be represented using any integer type, with false typically representing 0, and true typically representing 1. As an interesting side note, some CPUs are capable of comparing a register or memory location to 0 faster than 1, meaning that on some CPUs, it's actually faster for Boolean arithmetic and branching to result in false in the typical case.
Let's look at a bool using the following code:
#include <iostream>
int main(void)
{
auto num_bytes = sizeof(bool);
auto min = std::numeric_limits<bool>().min();
auto max = std::numeric_limits<bool>().max();
std::cout << "num bytes: " << num_bytes << '\n';
std::cout << "min value: " << min << '\n';
std::cout << "max value: " << max << '\n';
}
// > g++ scratchpad.cpp; ./a.out
// num bytes: 1
// min value: 0
// max value: 1
As shown in the preceding code, a Boolean using C++ on a 64 bit Intel CPU is 1 byte in size, and can take on a value of 0 or 1. It should be noted, for the same reasons as already identified, a Boolean could be 32-bits or even 64-bits, depending on the CPU architecture. On an Intel CPU, which is capable of supporting register sizes of 8 bits (that is, 1 byte), a Boolean only needs to be 1 byte in size.
The total size of a Boolean is important to note, with respect to storing Booleans in a file on disk. A Boolean technically only needs a single bit to store its value, but rarely (if any) CPU architectures support bit-style register and memory access, meaning a Boolean typically consumes more than a single bit, and in some cases could consume as many as 64 bits. If the size of your resulting file is important, storing a Boolean using the built-in Boolean type may not be preferred (ultimately resulting in the need for bit masking).