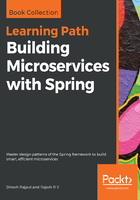
Creating auto searchable beans using Stereotype annotations
Let's see the following TransferService interface. Its implementation is annotated with @Component. Please refer to the following code:
package com.packt.patterninspring.chapter4.bankapp.service; public interface TransferService { void transferAmmount(Long a, Long b, Amount amount); }
The preceding interface is not important for this approach of configuration--I have taken it just for loose coupling in the application. Let's see its implementation, which is as follows:
package com.packt.patterninspring.chapter1.bankapp.service; import org.springframework.stereotype.Component; @Component public class TransferServiceImpl implements TransferService { @Override public void transferAmmount(Long a, Long b, Amount amount) { //business code here } }
You can see in the preceding code that TransferServiceImpl is annotated with the @Component annotation. This annotation is used to identify this class as a component class, which means, it is eligible to scan and create a bean of this class. Now there is no need to configure this class explicitly as a bean either by using XML or Java configuration--Spring is now responsible for creating the bean of the TransferServiceImpl class, because it is annotated with @Component.
As mentioned earlier, Spring provides us meta annotations for the @Component annotation as @Service, @Repository, and @Controller. These annotations are based on a specific responsibility at different layers of the application. Here, TransferService is the service layer class; as a best practice of Spring configuration, we have to annotate this class with the specific annotation, @Service, rather than with the generic annotation, @Component, to create the bean of this class. The following is the code for the same class annotated with the @Service annotation:
package com.packt.patterninspring.chapter1.bankapp.service; import org.springframework.stereotype.Service; @Service public class TransferServiceImpl implements TransferService { @Override public void transferAmmount(Long a, Long b, Amount amount) { //business code here } }
Let's see other classes in the application--these are the implementation classes of AccountRepository--and the TransferRepository interfaces are the repositories working at the DAO layer of the application. As a best practice, these classes should be annotated with the @Repository annotation rather than using the @Component annotation as shown next.
JdbcAccountRepository.java implements the AccountRepository interface:
package com.packt.patterninspring.chapter4.bankapp.repository.jdbc; import org.springframework.stereotype.Repository; import com.packt.patterninspring.chapter4.bankapp.model.Account; import com.packt.patterninspring.chapter4.bankapp.model.Amount; import com.packt.patterninspring.chapter4.bankapp.repository.
AccountRepository; @Repository public class JdbcAccountRepository implements AccountRepository { @Override public Account findByAccountId(Long accountId) { return new Account(accountId, "Arnav Rajput", new
Amount(3000.0)); } }
And JdbcTransferRepository.java implements the TransferRepository interface:
package com.packt.patterninspring.chapter4.bankapp.repository.jdbc; import org.springframework.stereotype.Repository; import com.packt.patterninspring.chapter4.bankapp.model.Account; import com.packt.patterninspring.chapter4.bankapp.model.Amount; import com.packt.patterninspring.chapter4.bankapp.
repository.TransferRepository; @Repository public class JdbcTransferRepository implements TransferRepository { @Override public void transfer(Account accountA, Account accountB, Amount
amount) { System.out.println("Transfering amount from account A to B via
JDBC implementation"); } }
In Spring, you have to enable component scanning in your application, because it is not enabled by default. You have to create a configuration Java file, and annotate it with @Configuration and @ComponentScan. This class is used to search out classes annotated with @Component, and to create beans from them.
Let's see how Spring scans the classes which are annotated with any stereotype annotations.