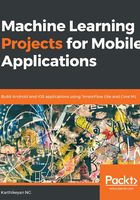
Core ML on an iOS app
Integrating Core ML on an iOS app is pretty straightforward. Go and download pre-trained models from the Apple developer page. Download MobileNet model from there.
After you download MobileNet.mlmodel, add it to the Resources group in your project. The vision framework eases our problems by converting our existing image formats into acceptable input types. You can see the details of your model as shown in the following screenshot. In the upcoming chapters, we will start creating our own models on top of existing models.
Let's look at how to load the model into our application:

Open ViewController.swift in your recently created Xcode project, and import both Vision and Core ML frameworks:
/**
Lets see the UIImage given to vision framework for the prediction.
The results could be slightly different based on the UIImage conversion.
**/
func visionPrediction(image: UIImage) {
guard let visionModel = try? VNCoreMLModel(for: model.model) else{
fatalError("World is gonna crash!")
}
let request = VNCoreMLRequest(model: visionModel) { request, error
in
if let predictions = request.results as? [VNClassificationObservation] {
//top predictions sorted based on confidence
//results come in string, double tuple
let topPredictions = observations.prefix(through: 5)
.map { ($0.identifier, Double($0.confidence)) }
self.show(results: topPredictions)
}
}
}
Let's load the same image through the Core ML MobileNet model for the prediction:
/**
Method that predicts objects from image using CoreML. The only downside of this method is, the mlmodel expects images in 224 * 224 pixels resolutions. So we need to manually convert UIImage
into pixelBuffer.
**/
func coremlPrediction(image: UIImage) {
if let makeBuffer = image.pixelBuffer(width: 224, height: 224),
let prediction = try? model.prediction(data: makeBuffer) {
let topPredictions = top(5, prediction.prob)
show(results: topPredictions)
}
}