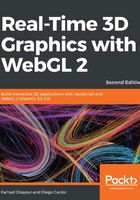
上QQ阅读APP看书,第一时间看更新
Defining JSON-Based 3D Models
Let's assume, for example, that we have a model object with two arrays: vertices and indices. Say that these arrays contain the information described in the cone example (ch02_06_cone.html), as follows:
function initBuffers() {
const vertices = [
1.5, 0, 0,
-1.5, 1, 0,
-1.5, 0.809017, 0.587785,
-1.5, 0.309017, 0.951057,
-1.5, -0.309017, 0.951057,
-1.5, -0.809017, 0.587785,
-1.5, -1, 0,
-1.5, -0.809017, -0.587785,
-1.5, -0.309017, -0.951057,
-1.5, 0.309017, -0.951057,
-1.5, 0.809017, -0.587785
];
indices = [
0, 1, 2,
0, 2, 3,
0, 3, 4,
0, 4, 5,
0, 5, 6,
0, 6, 7,
0, 7, 8,
0, 8, 9,
0, 9, 10,
0, 10, 1
];
// ...
}
Following the JSON notation, we would represent these two arrays as an object, as follows:
{
"vertices": [
1.5, 0, 0,
-1.5, 1, 0,
-1.5, 0.809017, 0.587785,
-1.5, 0.309017, 0.951057,
-1.5, -0.309017, 0.951057,
-1.5, -0.809017, 0.587785,
-1.5, -1, 0,
-1.5, -0.809017, -0.587785,
-1.5, -0.309017, -0.951057,
-1.5, 0.309017, -0.951057,
-1.5, 0.809017, -0.587785
],
"indices": [
0, 1, 2,
0, 2, 3,
0, 3, 4,
0, 4, 5,
0, 5, 6,
0, 6, 7,
0, 7, 8,
0, 8, 9,
0, 9, 10,
0, 10, 1
]
}
Based on this example, we can infer the following syntax rules:
- The extent of a JSON object is defined by curly brackets ({}).
- Attributes in a JSON object are separated by commas (,).
- There is no comma after the last attribute.
- Each attribute of a JSON object has two parts: a key and a value.
- The name of an attribute is enclosed by quotation marks ("").
- Each attribute key is separated from its corresponding value with a colon (:).
- Attributes of the array are defined in the same way you would define them in JavaScript.