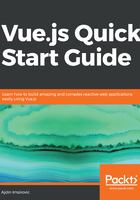
What is reactivity?
To grasp the concept better, let's look at an example code in which there is no reactivity. We will use an example that is very similar to the one we had in the previous chapter, when we were comparing Vue and vanilla JS. In the original example, using JavaScript, we created an unordered list and three list items inside of it. The values of the three list items were added from an array we declared, and the unordered list was populated with these list items using a for loop.
This time, we will do something slightly different. To see the example as a pen, visit https://codepen.io/AjdinImsirovic/pen/JZOZdR.
In this non-reactive example, we are predefining the members of the array as variables. Then we populate the array with those variables and print them to the screen as list items of an unordered list that gets appended to the document:
var a = 1;
var b = a + 1;
var c = b + 2;
var arr1 = [a,b,c];
var unorderedList = document.createElement('ul');
document.body.appendChild(unorderedList);
for (var i=0; i<3; i++) {
var listItem = document.createElement('li');
listItem.className = "list-item";
unorderedList.appendChild(listItem);
listItem.innerHTML = arr1[i];
}
arr1[0] = 2;
for (var i=0; i<3; i++) {
var listItem = document.createElement('li');
listItem.className = "list-item";
unorderedList.appendChild(listItem);
listItem.innerHTML = arr1[i];
}
However, what happens when we change a member of the array and repeat the for loop a second time? As we can see in the pen, the first and the fourth list items are different. The first value is 1, and the second value is 2. To make it more obvious, these items are in bold red text and have a gray background. The first value is the initial value of var a. The second value is the value of var a, updated with this line of code: arr1[0] = 2.
However, the values of variables b and c are not updated in the second for loop, even though we defined variables b and c in terms of variable a increased by 1 and 2, respectively.
So, we can see that there is no reactivity in JavaScript out of the box.
As far as Vue is concerned, reactivity is the term that is used to refer to the way in which Vue tracks changes. In other words, reactivity is the way in which changes in state are reflected in the DOM. Practically, this means that when a change is made to data, that change will be propagated to the page so that the user can see it. Therefore, saying that Vue is reactive is the same as saying Vue tracks changes. As a concept, it's as simple as that.