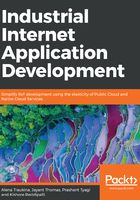
上QQ阅读APP看书,第一时间看更新
Edge Gateway triggering alerts
To run the Edge Gateway code, the prerequisites are given as follows:
- Download the code from GitHub. Samples are in the chapter 2 Iiot-Sample 1 project.
- The Edge Gateway code is in the folder iiot-edge-gateway.
- NPM and Node should be installed.
- To install the application, issue the following command:
npm install
- For this example, we are using socksjs as the client library for WebSocket. SocksJS provides the fallback between WebSockets and HTTP/HTTPS.
- Stomp protocol to send the data between the cloud and gateway.
WebSocket itself does not provide any message transfer protocol, hence using stomp over WebSocket solves that issue. Stomp is a generic protocol to send messages. In our example, we are using JSON to transfer the data.
- Details of the sample Edge Gateway is given in the following example. The code essentially reads data from a simulated sensor, in this case a .csv file, and then establishes connection to the server using the WebSocket protocol:
//include the stomp package and socks js
var Stomp = require('webstomp-client');
var SockJS = require('sockjs-client');
// reading CSV files using
var csv=require('fast-csv'),
fs = require("fs");
// open the connection to the WebSocket end point.
var socket = new SockJS('http://localhost:5074/iiot-sample-WebSocket');
//create a stomp session
var stompClient = Stomp.over(socket);
- Read from the CSV file and check for any deviation. Check for any reading above 50.
- If so, trigger an alert to the cloud service API.
- We will add security in the later chapters. This sample does not add authentication:
function readFromSensorDataAndCheckforAnomalies(){
var stream = fs.createReadStream("sensordata.csv");
csv
.fromStream(stream, {headers : true})
.validate(function(data){
return data.temp <= 50; //all reading under 50 are valid
})
.on("data-invalid", function(data){
var alertData = JSON.stringify({'alertsUuid':'alertsid'+ new Date(),
'severity':1,
'alertName':'HighTemprateAlert',
'alertInfo':'Temperature Value:' + data.temp });
stompClient.send("/app/createAlert",alertData,{});
})
.on("data", function(data){
console.log(data);
})
.on("end", function(){
console.log("done");
- Sample temperature data given in the following example should trigger two alerts to the cloud and it is contained in the sensordata.csv:
id,temp
1,5
2,50
3,400
4,20
5,50
6,70
- The JSON structure of the alerts that gets generated is given in the following example:
{
"alertsUuid": "alertsId",
"severity": 1,
"alertName": "HighTemperature Alert",
"alertInfo": "Temperature Value"
}
- Establish connection to the cloud service for generating alerts by subscribing to the alertCreated topic.
- Sending the alerts to the alerts service in the cloud is given as following (essentially we publish any WebSocket message to the cloud):
function createAlertInCloud() {
// Connect to cloud using WebSocket and create alerts
stompClient.connect({}, function (frame) {
//setConnected(true);
console.log('Connected: ' + frame);
stompClient.subscribe('/topic/alertCreated', function (alert) {
console.log('gotResponse from CloudService: ' + alert);
});
readFromSensorDataAndCheckforAnomalies();
});
};
createAlertInCloud();
- Run the node idiot-edge.js to see the result. It should send two alerts to the server:
node iiot-edge.js