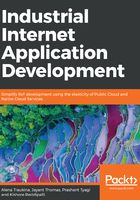
上QQ阅读APP看书,第一时间看更新
Running a receiver application on a PC
To run a receiver application on your PC, proceed as follows:
- Install and launch a PostgreSQL container:
docker run --rm --name postgres-container -e POSTGRES_PASSWORD=password -it -p 5433:5432 postgres
docker exec -it postgres-container createdb -U postgres iot-book
- Create the receiver folder.
- Create the ./receiver/package.json file with the following content:
{
"name": "receiver",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js",
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"pg": "^6.2.3"
}
}
- Create the ./receiver/index.js file with the following content, replacing the database credentials with the correct values:
var http = require('http');
var querystring = require('querystring');
var Pool = require('pg').Pool;
var pool = new Pool({
user: 'user',
database: 'database',
password: 'password',
host: 'host',
port: 5432
});
//ensure table exists in db
pool.query('CREATE TABLE IF NOT EXISTS "sensor-logs" (id serial NOT NULL PRIMARY KEY, data json NOT NULL)', function (err, result) {
if (err) console.log(err);
});
http.createServer(function (req, res) {
req.on('data', function (chunk) {
var data = querystring.parse(chunk.toString());
console.log(data);
//save in db
pool.query('INSERT INTO "sensor-logs" (data) VALUES ($1)', [data], function (err, result) {
if (err) console.log(err);
});
});
req.on('end', function () {
res.writeHead(200, 'OK', {'Content-Type': 'text/html'});
res.end('ok')
});
}).listen(process.env.PORT || 8080);
- Create the ./receiver/Dockerfile file with the following content:
FROM node:boron-onbuild
EXPOSE 8080
- Navigate to ./receiver.
- Build an image and run a Docker container:
# Build an image from a Dockerfile
docker build -t modbus-receiver .
# Run container in foreground
docker run -p 8080:8080 -it --rm --name modbus-receiver-container modbus-receiver
# Run container in background
# docker run -p 8080:8080 -d --rm --name modbus-receiver-container modbus-receiver
# Fetch the logs of a container
# docker logs -f modbus-sensor-container
# Stop running container
# docker stop modbus-receiver-container

Console output when a receiver app is running