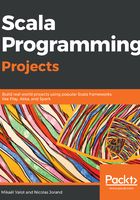
Declaring variables
In Scala, a variable can be declared using val or var. A val is immutable, which means you can never change its value. A var is mutable. It is not mandatory to declare the type of the variable. If you do not declare it, Scala will infer it for you.
Let's define some immutable variables:
scala> val x = 1 + 1
x: Int = 2
scala> val y: Int = 1 + 1
y: Int = 2
In both cases, the type of the variable is Int. The type of x was inferred by the compiler to be Int. The type of y was explicitly specified with : Int after the name of the variable.
We can define a mutable variable and modify it as follows:
scala> var x = 1
x: Int = 1
scala> x = 2
x: Int = 2
It is a good practice to use val in most situations. Whenever I see a val declared, I know that its content will never change subsequently. It helps to reason about a program, especially when multiple threads are running. You can share an immutable variable across multiple threads without fearing that one thread might see a different value at some point. Whenever you see a Scala program using var, it should make you raise an eyebrow: the programmer should have a good reason to use a mutable variable, and it should be documented.
If we attempt to modify a val, the compiler will raise an error message:
scala> val y = 1
y: Int = 1
scala> y = 2
<console>:12: error: reassignment to val
y = 2
^
This is a good thing: the compiler helps us make sure that no piece of code can ever modify a val.