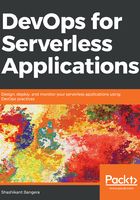
Lambda service and function deployment
In the following step, we will be create a simple Node.js service and Lambda function, and then deploy and invoke them:
- Create a new service using the Node.js template by Serverless Framework. We need to make sure that the name is unique and add the path to the service, which is optional. This command will create two files—handler.js and serverless.yml:
$ serverless create --template aws-nodejs --path my-serverless-service
Serverless: Generating boilerplate...
Serverless: Generating boilerplate in "/Users/shashi/Documents/packt/chapter2/serverless/my-serverless-service"
_______ __
| _ .-----.----.--.--.-----.----| .-----.-----.-----.
| |___| -__| _| | | -__| _| | -__|__ --|__ --|
|____ |_____|__| \___/|_____|__| |__|_____|_____|_____|
| | | The Serverless Application Framework
| | serverless.com, v1.26.1
-------'
Serverless: Successfully generated boilerplate for template: "aws-nodejs"
A service is the framework's unit of organization. It can be considered as a project file. It's where we can define functions, the events that trigger them, and the resources of the function that we will use. All of these are placed into one file serverless.yml. In this .yaml file, we define the service called my-serverless-service. Then we define the provider; as I mentioned earlier, Serverless Framework supports a lot of other cloud service providers. We can list the provider details in this tag and also mention the runtime, which in our case is Node.js. The runtime will change depending on the language we use to write the function. We can define the environment or stage we are deploying to—which in our case is dev—as well as the respective region. Then, in the functions section, we define the function name—which in our case is hello—which has an attribute called handler, and this handler will call the handler.js file. We can also define the memory size. Next, I have added an HTTP event, which, in addition to the Lambda function, provisions the AWS API Gateway. It will create and provide an endpoint for the handler. So, using one script, we can provision a Lambda function and an API endpoint. We can define various other attributes and parameters; we will look into these in more detail in the next chapter.
https://github.com/shzshi/my-serverless-service.git
$ cat serverless.yml
# Welcome to Serverless!
#
# This file is the main config file for your service.
# It's very minimal at this point and uses default values.
# You can always add more config options for more control.
# We've included some commented out config examples here.
# Just uncomment any of them to get that config option.
#
# For full config options, check the docs:
# docs.serverless.com
#
# Happy Coding!
service: my-serverless-service
# You can pin your service to only deploy with a specific Serverless version
# Check out our docs for more details
# frameworkVersion: "=X.X.X"
provider:
name: aws
runtime: nodejs6.10
stage: dev
region: us-east-1
functions:
hello:
handler: handler.hello
memorySize: 128
events:
- http:
path: handler
method: get
The handler.js code phrase is the Node.js Lambda function for Hello, World!, which is referenced in the serverless.yml file. It is a pretty simple function that will just display the message My Serverless World on execution, as shown in the following code:
$ cat handler.js
'use strict';
module.exports.hello = (event, context, callback) => {
const response = {
statusCode: 200,
body: JSON.stringify({
message: 'My Serverless World',
input: event,
}),
};
callback(null, response);
// Use this code if you don't use the http event with the LAMBDA-PROXY integration
// callback(null, { message: 'Go Serverless v1.0! Your function executed successfully!', event });
};