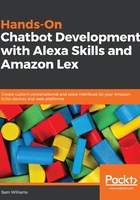
Writing your Lambda function's code
Once you've created your Lambda you are sent into the function editor within the Lambda Management Console. There is a lot going on this page, but we're focused on the section titled Function code.
When you first create a Lambda, it is created with a very basic function already implemented. This is nice as it gives you a starting point to build your function on. As we're using Node.js 8.10 as our runtime, there will be a single parameter of the event and then we will return our answer.
As a basic example, we'll create a Lambda that takes your name and age and tells you what your maximum heart rate is. This can be done more efficiently than the way we are going to do it, but this is done more as a way to demonstrate some techniques for use within Lambdas.
To start, we will console.log out the event and then extract the name and age. I'm going to use ES6 destructuring but you can also do this using normal variable declaration:
exports.handler = async (event) => {
console.log(event);
let { name, age } = event;
// same as => let name = event.name; let age = event.age
return 'Hello from Lambda!'
};
Now that we have the name and age from the event, we can pass those into a function that converts them into a string:
const createString = (name, age) => {
return `Hi ${name}, you are ${age} years old.`;
};
If you haven't seen this sort of string before, they're called template strings and they're much neater than previous string concatenation. Backticks start and end the string and you can insert data using ${data}.
Now we can change 'Hello from Lambda!' to createString(name, age) and our function will return our new string:
exports.handler = async (event) => {
console.log(event);
let { name, age } = event;
// same as => let name = event.name; let age = event.age
return createString(name, age);
};
Make sure to save your changes by clicking the bright orange Save button in the upper-right corner of your Lambda toolbar:
To test this out, we can click Test in the Lambda toolbar.
When we click on Test, a Configure test event popup opens. We can use this to decide what gets sent to our Lambda in the event payload. Give your test a name and then we can configure our data. For this Lambda it is very simple, just an object with keys of "name" and "age". Here's mine:
{
"name": "Sam",
"age": "24"
}
You can set your values to whatever you want and then click Save at the bottom of the configuration screen. Now the dropdown to the left of the Test button has changed to be your new test name. To run the test, simply click the Test button:
If your response is still 'Hello from Lambda!', then make sure you've saved your function and run the test again. As you can see, I got the response of "Hi Sam, you are 24 years old.", which is what we expected. As well as the response, we get a RequestID and Function Logs. Remember when we added that console.log(event) to our code? You can now see that the object { name: 'Sam', age: '24' } was logged out. If you want to see more of your logs or logs from previous Lambda calls, they're all stored in CloudWatch. To get to CloudWatch you can either search for it in the services or get there by selecting Monitoring at the top of the Lambda Console and then clicking View logs in CloudWatch.
There are also some interesting graphs inside Monitoring that can tell you a lot about how well your function is working:
Lambda functions can be created in a single file like we have done, but they also work with multiple files. When your Lambda is doing very complex tasks, you can break each section out into its own file to improve organization and readability.
We're going to create a new file called hr.js, and inside we're going to create and export another function. This function is going to calculate your maximum heart rate based on your age. Create the new file by right-clicking in the folder menu and selecting New File and call it hr.js. Open up that file and we'll create a calculateHR function:
module.exports = {
calculateHR: (age) => {
return 220 - age;
}
}
Now, back in our index.js file we need to import our hr.js file and call the calculateHR function:
const HR = require('./hr');
exports.handler = async (event) => {
console.log(event);
let { name, age } = event;
return createString(name, age);
};
const createString = (name, age) => {
return `Hi ${name}, you are ${age} years old and have a maximum heart rate of ${HR.calculateHR(age)}.`;
};
When we run our last test again, we get a new response of "Hi Sam, you are 24 years old and have a maximum heart rate of 196.". This could have been done a lot more effectively, but this was done more to show you some ways that you can write code in Lambda functions.