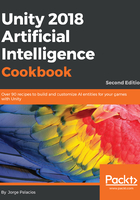
上QQ阅读APP看书,第一时间看更新
Getting ready
We need to create a basic matching-velocity algorithm and the notion of jump pads and landing pads in order to emulate velocity math so that we can reach them.
The following is the code for the VelocityMatch behavior:
using UnityEngine; using System.Collections; public class VelocityMatch : AgentBehaviour { public float timeToTarget = 0.1f; public override Steering GetSteering() { Steering steering = new Steering(); steering.linear = target.GetComponent<Agent>().velocity - agent.velocity; steering.linear /= timeToTarget; if (steering.linear.magnitude > agent.maxAccel) steering.linear = steering.linear.normalized * agent.maxAccel; steering.angular = 0.0f; return steering; } }
Also, it's important to create a data type called JumpPoint:
using UnityEngine; using System.Collections; public class JumpPoint { public Vector3 jumpLocation; public Vector3 landingLocation; //The change in position from jump to landing public Vector3 deltaPosition; public JumpPoint () : this (Vector3.zero, Vector3.zero) { } public JumpPoint(Vector3 a, Vector3 b) { this.jumpLocation = a; this.landingLocation = b; this.deltaPosition = this.landingLocation - this.jumpLocation; } }