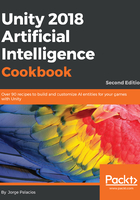
上QQ阅读APP看书,第一时间看更新
How to do it...
Thanks to our previous hard work, this recipe is a short one:
- Create the AvoidWall behavior derived from Seek:
using UnityEngine; using System.Collections; public class AvoidWall : Seek { // body }
- Include the member variables for defining the safety margin and the length of the ray to cast:
public float avoidDistance; public float lookAhead;
- Define the Awake function to set up the target:
public override void Awake() { base.Awake(); target = new GameObject(); }
- Define the GetSteering function required for future steps:
public override Steering GetSteering() { // body }
- Declare and set the variable needed for ray casting:
Steering steering = new Steering(); Vector3 position = transform.position; Vector3 rayVector = agent.velocity.normalized * lookAhead; Vector3 direction = rayVector; RaycastHit hit;
- Cast the ray and make the proper calculations if a wall is hit:
if (Physics.Raycast(position, direction, out hit, lookAhead)) { position = hit.point + hit.normal * avoidDistance; target.transform.position = position; steering = base.GetSteering(); } return steering;