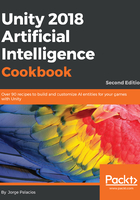
上QQ阅读APP看书,第一时间看更新
How to do it...
Now, we need to make some changes to the Agent class:
- Add a new namespace from the library:
using System.Collections.Generic;
- Add the member variable for the minimum steering value to consider a group of behaviors:
public float priorityThreshold = 0.2f;
- Add the member variable for holding the group of behavior results:
private Dictionary<int, List<Steering>> groups;
- Initialize the variable in the Start function:
groups = new Dictionary<int, List<Steering>>();
- Modify the LateUpdate function so that the steering variable is set by calling GetPrioritySteering:
public virtual void LateUpdate () { // funnelled steering through priorities steering = GetPrioritySteering(); groups.Clear(); // ... the rest of the computations stay the same steering = new Steering(); }
- Modify the SetSteering function's signature and definition to store the steering values in their corresponding priority groups:
public void SetSteering (Steering steering, int priority) { if (!groups.ContainsKey(priority)) { groups.Add(priority, new List<Steering>()); } groups[priority].Add(steering); }
- Finally, implement the GetPrioritySteering function to funnel the steering group:
private Steering GetPrioritySteering () { Steering steering = new Steering(); float sqrThreshold = priorityThreshold * priorityThreshold; foreach (List<Steering> group in groups.Values) { steering = new Steering(); foreach (Steering singleSteering in group) { steering.linear += singleSteering.linear; steering.angular += singleSteering.angular; } if (steering.linear.sqrMagnitude > sqrThreshold || Mathf.Abs(steering.angular) > priorityThreshold) { return steering; } } return steering; }