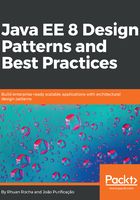
上QQ阅读APP看书,第一时间看更新
Implementing the entity with JPA
The JPA entity is a class that represents some table or view of a database. The entity needs to have an attribute that identifies only one entity, needs to have a constructor with non-arguments and each object of a class that is a JPA entity identify only one row of table or view.
In the following code, we have an interface, called Entity, that all JPA entities will implement according to the following method:
public interface Entity < T > {
public T getId();
}
In the following code, we have the transfer object, called Employee, which is a JPA entity. This class has the mapping table, called Employee, as well as its column used by applications. The business tier only needs to know the transfer objects, the DAOs, and the parameters to send to the DAOs:
import javax.persistence.*;
import javax.validation.constraints.NotNull;
import java.util.Objects;
@javax.persistence.Entity(name = "Employee")
public class Employee implements Entity<Long> {
@Id
@GeneratedValue
@Column(name = "id")
private Long id;
@NotNull
@Column(name="name")
private String name;
@Column(name="address")
private String address;
@NotNull
@Column(name="salary")
private Double salary;
public Employee(){}
public Employee( String name, String address, Double salary){
this.name = name;
this.address = address;
this.salary = salary;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public Double getSalary() {
return salary;
}
public void setSalary(Double salary) {
this.salary = salary;
}
@Override
public Long getId() {
return this.id;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Employee employee = (Employee) o;
return Objects.equals(id, employee.id);
}
@Override
public int hashCode() {
return Objects.hash(id);
}
}