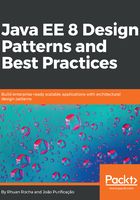
Implementing the domain-store pattern
This is a very long pattern. To implement and facilitate this implementation, we will save all the data on HashMap. This is an important step, because the focus of this subsection is to demonstrate how to implement domain-store patterns. To facilitate our understanding, we will look at the same scenario covered in the Implementing the data-access object pattern subsection. Here, we have the transfer object, called Employee, and we will also read and write data on the data source. However, persistence will be oriented by object state and will have an intelligence in its logic.. In this implementation, we have the following classes, interfaces, and annotations:
- PersistenceManagerFactory: This works as a factory pattern and is responsible for creating instances of the PersistenceManager class. PersistenceManagerFactory is a singleton and has only one instance on the entire application.
- PersistenceManager: This manages the persistence and queries the data. This data is an object model that works as a Unit of Work.
- Persistence: This is an annotation used as the qualify of CDI. This qualify is used to define a method of PersistenceManagerFactory, which is responsible for creating a PersistenceManager instance.
- EmployeeStoreManager: This works as a Data-Access Object (DAO), interacting with the data source and encapsulating all the data source complexity. DAO is responsible for reading and writing employee data on the data source.
- StageManager: This is an interface used to create all the StageManager implementations.
- EmployeeStageManager: This coordinates the read and writes operations of data according to its states and rules.
- TransactionFactory: This works as a factory pattern and is responsible for creating Transaction instances. TransactionFactory is a singleton and has only one instance in the entire application.
- Transaction: This is used to create transaction-oriented policies. This class controls the life cycle of transactions and defines the transaction limits.
- Transaction (annotation): Annotation used as the qualify of CDI. This qualify is used to define a method of TransactionFactory that is responsible for creating a Transaction instance.
To implement this pattern, we will begin with the PesistenceManagerFactory class, which is a factory of PersistenceManager.