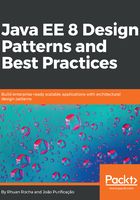
上QQ阅读APP看书,第一时间看更新
Implementing the EmployeeBusiness class
The EmployeeBusiness class has the employee business logic:
import javax.ejb.Stateless;
import javax.inject.Inject;
import java.util.Optional;
@Stateless
public class EmployeeBusiness{
@Inject @Persistence
protected PersistenceManager persistenceManager;
public boolean save ( Employee employee ) throws Exception {
//Begin Transaction
persistenceManager.begin();
persistenceManager.persist(employee);
//End Transaction
persistenceManager.commit();
return true;
}
public Optional<Employee> findById(Employee employee ){
return Optional.ofNullable( (Employee) persistenceManager.load(employee.getId()).get());
}
}
In the preceding code block, we have the EmployeeBusiness class, which has the employee business logic. This class has the save(Employee) method, which is used to save employee data, and findById(Employee ), which is used to find an employee according to their ID. Note that, on the save method, we call the begin() method as well as commit() from the PersistenceManager class. These calls define the delimiting of a transaction and only the data is saved at the data source when the commit() method is called.