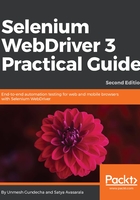
Understanding frozen preferences
Now, let's go back to the big list of frozen preferences that user.js contains, which we saw earlier. The Firefox Driver thinks that a test-script developer doesn't have to deal with them and doesn't allow those values to be changed. Let's pick one frozen preference and try to change its values in our code. Let's consider the browser.shell.checkDefaultBrowser preference, whose value FirefoxDriver implementers thought should be set to false so that the Firefox browser does not ask you whether to make Firefox your default browser, if it is not already, when you are busy executing your test cases. Ultimately, you don't have to deal with the pop-up itself in your test scripts. Apart from setting the preference value to false, the implementers of FirefoxDriver also thought of freezing this value so that users don't alter these values. That is the reason these preferences are called frozen preferences. Now, what happens if you try to modify these values in your test scripts? Let's see a code example:
public class FirefoxFrozenPreferences {
public static void main(String... args) {
System.setProperty("webdriver.gecko.driver",
"./src/test/resources/drivers/geckodriver 2");
FirefoxProfile profile = new FirefoxProfile();
profile.setPreference("browser.shell.checkDefaultBrowser", true);
FirefoxOptions firefoxOptions = new FirefoxOptions();
firefoxOptions.setProfile(profile);
FirefoxDriver driver = new FirefoxDriver(firefoxOptions);
driver.get("http://facebook.com");
}
}
Now when you execute your code, you will immediately see an exception saying you're not allowed to override these values. The following is the exception stack trace you will see:
Exception in thread "main" java.lang.IllegalArgumentException: Preference browser.shell.checkDefaultBrowser may not be overridden: frozen value=false, requested value=true
This is how FirefoxDriver mandates a few preferences that are not to be touched. However, there are a few preferences of the frozen list that FirefoxDriver allows to alter through code. For that, it explicitly exposes methods in the FirefoxProfile class. Those exempted preferences are for dealing with SSL certificates and native events. Here, we will see how we can override the SSL certificates' preferences.
Let's use a code example that tries to override the default Firefox behavior to handle SSL certificates. The FirefoxProfile class has two methods to handle the SSL certificates; the first one is as follows:
public void setAcceptUntrustedCertificates(boolean acceptUntrustedSsl)
This lets Firefox know whether to accept SSL certificates that are untrusted. By default, it is set to true, that is, Firefox accepts SSL certificates that are untrusted. The second method is as follows:
public void setAssumeUntrustedCertificateIssuer(boolean untrustedIssuer)
This lets Firefox assume that the untrusted certificates are issued by untrusted or self-signed certification agents. Firefox, by default, assumes the issuer to be untrusted. That assumption is particularly useful when you test an application in the test environment while using the certificate from the production environment.
The preferences, webdriver_accept_untrusted_certs and webdriver_assume_ untrusted_issuer, are the ones related to the SSL certificates. Now, let's create a Java code to modify the values for these two values. By default, the values are set to true, as seen in the user.js file. Let's mark them as false with the following code:
public static void main(String... args) {
System.setProperty("webdriver.gecko.driver",
"./src/test/resources/drivers/geckodriver 2");
FirefoxProfile profile = new FirefoxProfile();
profile.setAssumeUntrustedCertificateIssuer(false);
profile.setAcceptUntrustedCertificates(false);
FirefoxOptions firefoxOptions = new FirefoxOptions();
firefoxOptions.setProfile(profile);
FirefoxDriver driver = new FirefoxDriver(firefoxOptions);
driver.get("http://facebook.com");
}
Here, we have set the values to false, and now if we open the user.js file in the profile directory of this instance of Firefox, you will see the values set to false, as follows:
user_pref("webdriver_accept_untrusted_certs", false);
user_pref("webdriver_assume_untrusted_issuer", false);