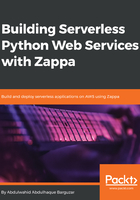
A minimal Flask application
Let's see what a minimal Flask application looks like:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return 'Hello World!'
That's it, we are done with the minimal Flask application. It's very simple to configure and create a microservice with Flask.
Let's discuss what exactly the preceding code is doing and how we would run the program:
- First, we imported a Flask class.
- Next, we created an instance of the Flask class. This instance will be our WSGI application. This first argument will be the name of the module or package. Here, we created a single module, hence we used __name__. This is needed so that Flask knows where to look for templates, static, and other directories.
- Then, we used app.route as a decorator with a URL name as a parameter. This will define and map the route with the specified function.
- The function will be invoked to the HTTP request with the URL specified in the route decorator.
To run this program, you can either use the flask command or python -m flask, but before that, you need to set an environment variable as FLASK_APP with a module file name of where the Flask instance was defined:
$ export FLASK_APP=hello_world.py
$ flask run
* Serving Flask app "flask_todo.hello_world"
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
This launches a built-in server that is good enough for testing and debugging locally. The following is a screenshot of the localhost running in the browser:

Of course, it wouldn't work with production, but Flask provides numerous options for deployment. You can have a look at http://flask.pocoo.org/docs/0.12/deploying/#deployment for more information, but in our case, we are going to deploy to a serverless environment on AWS Lambda and API Gateway using Zappa.