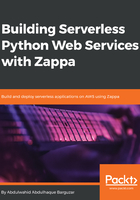
上QQ阅读APP看书,第一时间看更新
Forms
Flask-WTF extensions provide the ability to develop forms in Flask and render them through Jinja2 templates. Flask-WTF extends the WTForms library, which has standard patterns to design a form.
We need two forms for this, such as SignupForm and LoginForm. The following is the code for creating forms classes.
File—auth/forms.py:
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, SubmitField
from wtforms.validators import Required, Length, Email, EqualTo
class LoginForm(FlaskForm):
email = StringField(
'Email', validators=[Required(), Length(1,64), Email()]
)
password = PasswordField(
'Password', validators=[Required()]
)
submit = SubmitField('Log In')
class SignupForm(FlaskForm):
email = StringField(
'Email', validators=[Required(), Length(1,64), Email()]
)
password = PasswordField(
'Password', validators=[
Required(),
EqualTo('confirm_password', message='Password must match.')]
)
confirm_password = PasswordField(
'Confirm Password', validators=[Required()]
)
submit = SubmitField('Sign up')
Here, we create the forms with some validations. Now, we are going to use these forms in the views section, where we are going to render the templates along with the forms context.