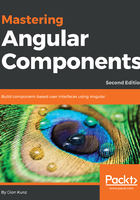
Creating a task list
Now that we have our main application component set up, we can go on and start fleshing out our task management application. The second component that we're going to create will be responsible for listing tasks. Following the concept of composition, we'll create a task list component as a subcomponent of our main application component.
Let's create a new component for our task list by using the Angular CLI generator functionality. We already want to structure our application by area, where we put all task-relevant components into a tasks subfolder:
ng generate component --spec false -ve none tasks/task-list
Using the --spec false option while generating our component, we can skip creating test specifications. Since we're going to cover testing in a later chapter, we're skipping this process for the moment. Also, by using the -ve none parameter, we can tell Angular to create the component using ViewEncapsulation.None as a default encapsulation setting.
Let's open the generated file src/app/tasks/task-list.ts and do some modifications to it:
import {Component, ViewEncapsulation} from '@angular/core'; @Component({ selector: 'mac-task-list',
templateUrl: './task-list.component.html',
encapsulation: ViewEncapsulation.None
})
export class TaskListComponent {
tasks = [
{id: 1, title: 'Task 1', done: false},
{id: 2, title: 'Task 2', done: true}
];
}
We've created a very simple task list component that has a list of tasks stored internally. This component will be attached to HTML elements that match the CSS element selector mac-task-list.
Now, let's create a view template for this component to display the tasks. As you can see from the templateUrl property within the component metadata, we are looking for a file called task-list.component.html.
Let's change the content of this file to match the following excerpt:
<div *ngFor="let task of tasks"> <input type="checkbox" [checked]="task.done"> <div>{{task.title}}</div> </div>
We use the NgFor directive to repeat the outermost DIV element for as many tasks as we have on the task list of our component. The NgFor directive in Angular will create a template element from its underlying content and instantiate as many elements from the template as the expression evaluates to. We currently have two tasks in our task list component, so this will create two instances of our template.
All that's left to do in order to make our task list work is include the task list component within the main application component. We can go ahead and modify our src/app/app.component.html file and change its content to match the following:
<mac-task-list></mac-task-list>
This was the last change we needed to make in order to make our task list component work. To view your changes, you can start the serving mode of Angular CLI, if you don't have it running already.