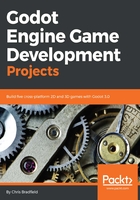
Adding the HUD to Main
Now, you need to set up the communication between the Main scene and the HUD. Add an instance of the HUD scene to the Main scene. In the Main scene, connect the timeout() signal of GameTimer and add the following:
func _on_GameTimer_timeout():
time_left -= 1
$HUD.update_timer(time_left)
if time_left <= 0:
game_over()
Every time the GameTimer times out (every second), the remaining time is reduced.
Next, connect the pickup() and hurt() signals of the Player:
func _on_Player_pickup():
score += 1
$HUD.update_score(score)
func _on_Player_hurt():
game_over()
Several things need to happen when the game ends, so add the following function:
func game_over():
playing = false
$GameTimer.stop()
for coin in $CoinContainer.get_children():
coin.queue_free()
$HUD.show_game_over()
$Player.die()
This function halts the game, and also loops through the coins and removes any that are remaining, as well as calling the HUD's show_game_over() function.
Finally, the StartButton needs to activate the new_game() function. Click on the HUD instance and select its new_game() signal. In the signal connection dialog, click Make Function to Off and in the Method In Node field, type new_game. This will connect the signal to the existing function rather than creating a new one. Take a look at the following screenshot:

Remove new_game() from the _ready() function and add these two lines to the new_game() function:
$HUD.update_score(score)
$HUD.update_timer(time_left)
Now, you can play the game! Confirm that all the parts are working as intended: the score, the countdown, the game ending and restarting, and so on. If you find a piece that's not working, go back and check the step where you created it, as well as the step(s) where it was connected to the rest of the game.