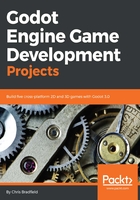
What is a tween?
A tween is a way to interpolate (change gradually) some value over time (from a start value to an end value) using a particular function. For example, you might choose a function that steadily changes the value or one that starts slow but ramps up in speed. Tweening is also sometimes referred to as easing.
When using a Tween node in Godot, you can assign it to alter one or more properties of a node. In this case, you're going to increase the Scale of the coin and also cause it to fade out using the Modulate property.
Add this line to the _ready() function of Coin:
$Tween.interpolate_property($AnimatedSprite, 'scale',
$AnimatedSprite.scale,
$AnimatedSprite.scale * 3, 0.3,
Tween.TRANS_QUAD,
Tween.EASE_IN_OUT)
The interpolate_property() function causes the Tween to change a node's property. There are seven parameters:
- The node to affect
- The property to alter
- The property's starting value
- The property's ending value
- The duration (in seconds)
- The function to use
- The direction
The tween should start playing when the player picks up the coin. Replace queue_free() in the pickup() function:
func pickup():
monitoring = false
$Tween.start()
Setting monitoring to false ensures that the area_enter() signal won't be emitted if the player touches the coin during the tween animation.
Finally, the coin should be deleted when the animation finishes, so connect the Tween node's tween_completed() signal:
func _on_Tween_tween_completed(object, key):
queue_free()
Now, when you run the game, you should see the coins growing larger when they're picked up. This is good, but tweens are even more effective when applied to multiple properties at once. You can add another interpolate_property(), this time to change the sprite's opacity. This is done by altering the modulate property, which is a Color object, and changing its alpha channel from 1 (opaque) to 0 (transparent). Refer to the following code:
$Tween.interpolate_property($AnimatedSprite, 'modulate',
Color(1, 1, 1, 1),
Color(1, 1, 1, 0), 0.3,
Tween.TRANS_QUAD,
Tween.EASE_IN_OUT)