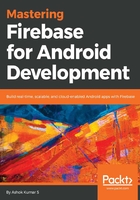
Finalizing the project
Now, let's write the code for Sign in or Sign up using an Email provider. Already signed up users can enter the email ID and password to start using the application. In the case of new account creation, users should be able to enter the necessary information in the form to create an account. When we use the FirebaseUI library, the bare minimum UI is taken care of. The following code dictates the steps for creating email onboarding:
// Add this in global scope
private static final int REQUEST_CODE = 101;
private void Authenticate() {
startActivityForResult(
AuthUI.getInstance().createSignInIntentBuilder()
.setAvailableProviders(getAuthProviderList())
.setIsSmartLockEnabled(false)
.build(),
REQUEST_CODE);
}
private List<AuthUI.IdpConfig> getAuthProviderList() {
List<AuthUI.IdpConfig> providers = new ArrayList<>();
providers.add(
new AuthUI.IdpConfig.Builder(AuthUI.EMAIL_PROVIDER).build());
return providers;
}
The getauthproviderlist method returns the email provider among all the other providers. So, we have set up the required line of codes to onboard, but we have a few more things to ensure, The Authenticate method starting an activity for the result this needs to be handled like shown in the following:
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
IdpResponse response = IdpResponse.fromResultIntent(data);
if (requestCode == REQUEST_CODE) {
if (resultCode == ResultCodes.OK) {
startActivity(new Intent(this, DashBoardActivity.class));
return;
}
} else {
if (response == null) {
// if user cancelled Sign-in
return;
}
if (response.getErrorCode() == ErrorCodes.NO_NETWORK) {
// When device has no network connection
return;
}
if (response.getErrorCode() == ErrorCodes.UNKNOWN_ERROR) {
// When unknown error occurred
return;
}
}
}
In DashBoardActivity, we need to show who logged in. We can use a simple Toast message to show this information:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
.....
FirebaseUser currentUser =
FirebaseAuth.getInstance().getCurrentUser();
if (currentUser == null) {
startActivity(new Intent(this, MainActivity.class));
finish();
return;
}
Toast.makeText(this, " "+currentUser.getEmail()+ currentUser.getDisplayName(),
Toast.LENGTH_SHORT).show();
}
To sign out manually, we can use the following method, which makes use of AuthUI. Here, the AuthUI class will reach out to the Firebase servers to check the instance. We will subscribe to the signOut method, and then we will attach addOnCompleteListener to return the task of log in success or failure:
public void signOut(View view) {
AuthUI.getInstance()
.signOut(this)
.addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull Task<Void> task) {
if (task.isSuccessful()) {
startActivity(new Intent(
DashBoardActivity.this,
MainActivity.class));
finish();
} else {
// Report error to user
}
}
});
}
If your project needs account removal, AuthUI provides all the required details. We will then have to subscribe to the delete method and attach the addOnCompleteListener callback to check the task for successful account removal:
public void removeAccount(View view) {
AuthUI.getInstance()
.delete(this)
.addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull Task<Void> task) {
if (task.isSuccessful()) {
startActivity(new Intent(DashBoardActivity.this,
MainActivity.class));
finish();
} else {
// Notify user of error
}
}
});
}
We have now completed exploring Firebase authentication for email providers using Firebase auth UI. In the following section, let's see how to achieve without using any of the libraries; in other words, when we need the ability to customize the UI for the authentication system.