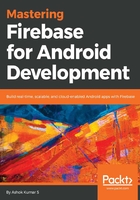
Twitter sign-in
Firebase user authentication can also be performed using the Twitter SDK. Similar to Google and Facebook integration, the user is told to sign in to a Twitter account, the points of interest of which are then used to create an account in the Firebase authentication user database.
We need to enable the Twitter sign in method in Firebase console. Add the appropriate new Gradle dependency to the project. Since we are using the Firebase SDK, we will have control over creating the user interface. But, we are focusing on the main aspects here.
The Twitter SDK also provides the button designed for Android:
<com.twitter.sdk.android.core.identity.TwitterLoginButton
android:id="@+id/loginButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintBottom_toTopOf="@+id/button3"
app:layout_constraintTop_toBottomOf="@+id/statusText" />
Before loading the layout inside the oncreate method, initialize Twitter:
TwitterConfig config = new TwitterConfig.Builder(this)
.logger(new DefaultLogger(Log.DEBUG))
.twitterAuthConfig(new TwitterAuthConfig(TWITTER_KEY,
TWITTER_SECRET))
.debug(true)
.build();
Twitter.initialize(config);
Using Twitter button callbacks, we can have a reference to the success or failure of user data retrieval:
loginButton.setCallback(new Callback<TwitterSession>() {
@Override
public void success(Result<TwitterSession> result) {
Log.d(TAG, "loginButton Callback: Success");
}
@Override
public void failure(TwitterException exception) {
Log.d(TAG, "loginButton Callback: Failure " +
exception.getLocalizedMessage());
}
});
Now, we can use Firebase to create the account using the Twitter profile details:
private void TwitterFirebase(TwitterSession session) {
AuthCredential credential = TwitterAuthProvider.getCredential(
session.getAuthToken().token,
session.getAuthToken().secret);
FirebaseAuth.signInWithCredential(credential)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (!task.isSuccessful()) {
Log.w(TAG, "signInWithCredential",
task.getException());
Toast.makeText(TwitterAuthActivity.this,
"Authentication failed.",
Toast.LENGTH_SHORT).show();
}
}
});