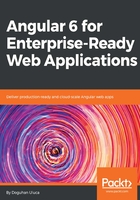
Angular unit tests
Just because your Angular app launches using npm start and seems to work fine, it doesn't mean it is error free or production ready. As covered earlier in Chapter 2, , Angular CLI creates a unit test file as you create new components and services, such as current-weather.component.spec.ts and weather.service.spec.ts.
At their most basic, these unit default unit tests ensure that your new components and services can be properly instantiated in the test harness. Take a look at the following spec file and observe the should create test. The framework asserts that component of the CurrentWeatherComponent type to not be null or undefined, but be truthy:
src/app/current-weather/current-weather.component.spec.ts
describe('CurrentWeatherComponent', () => {
let component: CurrentWeatherComponent
let fixture: ComponentFixture<CurrentWeatherComponent>
beforeEach(
async(() => {
TestBed.configureTestingModule({
declarations: [CurrentWeatherComponent],
}).compileComponents()
})
)
beforeEach(() => {
fixture = TestBed.createComponent(CurrentWeatherComponent)
component = fixture.componentInstance
fixture.detectChanges()
})
it('should create', () => {
expect(component).toBeTruthy()
})
})
The WeatherService spec contains a similar test. However, you'll note that both types of tests are set up slightly differently:
src/app/weather/weather.service.spec.ts
describe('WeatherService', () => {
beforeEach(() => {
TestBed.configureTestingModule({
providers: [WeatherService],
})
})
it('should be created', inject([WeatherService], (service: WeatherService) => {
expect(service).toBeTruthy()
})
)
})
In the WeatherService spec's beforeEach function, the class under test is being configured as a provider and then injected into the test. On the other hand, the CurrentWeatherComponent spec has two beforeEach functions. The first, the beforeEach function declares and compiles the component's dependent modules asynchronously, while the second, the beforeEach function creates a test fixture and starts listening to changes in the component, ready to run the tests once the compilation is complete.