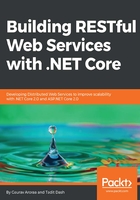
Interesting facts
Let's experiment with a few things now. You will get a very good insight into what happens behind the scenes:
- Add another method, Get12(), to the controller and remove the [HttpGet] method:
public IEnumerable<string> Get12()
{
return new string[] { "value1", "value2", "value3" };
}
// GET api/values
//[HttpGet] - Remove this attribute
public IEnumerable<string> Get()
{
return new string[] { "value1", "value2" };
}
What do you think the output would be? It's interesting. Here is the output:

That means it found two GET methods and it was not able to decide which one to execute. Note that neither of them is decorated by an attribute, such as [HttpGet].
- Now let's plan to bring back the attribute and test what happens. However, we will decorate the new Get12 method and leave the old Get method intact with the attribute commented. So, the code would be:
[HttpGet]
public IEnumerable<string> Get12()
{
return new string[] { "value1", "value2", "value3" };
}
// GET api/values
//[HttpGet]
public IEnumerable<string> Get()
{
return new string[] { "value1", "value2" };
}
Let's have a quick look at what we did to the output:

Clear enough! The Get12 method was executed and the reason for this was that we explicitly told it that it was the Get method by way of the [HttpGet] attribute.
- More fun can be experienced by adding an attribute to both of the methods:
[HttpGet]
public IEnumerable<string> Get12()
{
return new string[] { "value1", "value2", "value3" };
}
// GET api/values
[HttpGet]
public IEnumerable<string> Get()
{
return new string[] { "value1", "value2" };
}
Can you guess the output? Yes, it is the same as we saw when we had both methods without the attribute AmbiguousActionException as shown in the following screenshot:

- Finally, let's have another method named HelloWorld() with the attribute along with the existing ones. Let's remove the attributes from the other ones:
[HttpGet]
public string HelloWorld()
{
return "Hello World";
}
public IEnumerable<string> Get12()
{
return new string[] { "value1", "value2", "value3" };
}
// GET api/values
public IEnumerable<string> Get()
{
return new string[] { "value1", "value2" };
}
Perfect! Let's see the output. It's Hello World in the browser:
