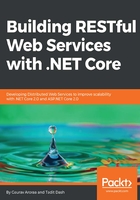
GET
I will add another controller called ProductsController. For now, let's have a simple action method, GET, which will return some products. The products are hard-coded in the action method for now. The method will look like the following:
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
namespace DemoECommerceApp.Controllers
{
[Produces("application/json")]
[Route("api/[Controller]")]
public class ProductsController : Controller
{
// GET: api/Products
[HttpGet]
public IEnumerable<Product> Get()
{
return new Product[]
{
new Product(1, "Oats", new decimal(3.07)),
new Product(2, "Toothpaste", new decimal(10.89)),
new Product(3, "Television", new decimal(500.90))
};
}
}
}
The [Route] attribute is provided with a well-defined template of "api/[Controller]". Here, the controller name is ProductsController. When we request using the URL api/Products, the framework will search for a controller with that route defined on it. The [Controller] placeholder is a special naming convention that will be replaced with the text (name of controller) Products at runtime. However, you can directly write the fully qualified template with the controller name, such as [Route (api/Products)].
So, this GET method will return us three products with their details. The Product class can be designed, like the following, with a constructor to build Product objects:
public class Product
{
public Product(int id, string name, decimal price)
{
Id = id;
Name = name;
Price = price;
}
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
We are done. Let's do a GET request through Postman to analyze the request and response mechanism in REST. For a GET request, it's simple. Just open Postman. Then follow the steps mentioned in the following screenshot:

Executing GET request in Postman
In Step-1, just paste the URL, which is http://localhost:57571/api/products for our example. Everything else is already set for a GET request. You can see the request type to the left of the URL box, which is GET. That means the current request will be a GET request. Hit the Send button as shown in Step-2.
The response is a list of products shown inside the section at the bottom. It's in .json format. Please refer to the following screenshot, which displays the response of the GET request:

Now that you have had a pleasant look at how GET works, let's analyze what happens behind the scenes. The client, which is Postman here, sends an HTTP request and gets a response in return. While sending requests it also specifies the Request Headers, and the server, in return, sends the Response Headers.
HTTP Headers enable the client and server to both send and receive additional information with the request and response respectively. This decides the exact behavior of the HTTP transaction. You can refer to the following resources to learn more about the headers. We will have a quick look at the headers in the next section:
- https://www.w3.org/Protocols/rfc2616/rfc2616-sec14.html
- https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers
In Postman, you can click on Code, as shown in the following screenshot:

Clicking on this link will open one modal that will show you the HTTP Request Headers sent to the server to serve the request. Check out the following screenshot of the modal, which clearly mentions the Request Type as GET, Host as the URL of the API, and then other headers such as Cache-Control and Postman-Token:

Want to know what the jQuery code snippet looks like for this GET call? It's super-easy with Postman. Click on Code on the main screen, then from the drop-down menu containing languages, select jQuery. (See the following screenshot.) Moreover, you can get code in different languages by selecting from the drop-down list. Happy copying!

Response Header is clearly shown on the main page, as shown in the following screenshot. Notice that there is a Status code mentioned, which is 200 OK in this case. So, what does this code signify?
Let's talk about it in the next section.

Postman Response Headers