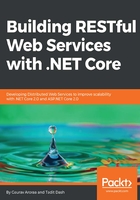
上QQ阅读APP看书,第一时间看更新
Configuring DbContext
The context class can be found in the same folder that has the OnConfiguring and OnModelCreating methods with a property for Customers.
The following code block shows the FlixOneStoreContext class:
using Microsoft.EntityFrameworkCore;
namespace DemoECommerceApp.Models
{
public partial class FlixOneStoreContext : DbContext
{
public virtual DbSet<Customers> Customers { get; set; }
public FlixOneStoreContext(DbContextOptions<
FlixOneStoreContext> options)
: base(options)
{ }
// Code is commented below, because we are applying
dependency injection inside startup.
// protected override void OnConfiguring(
DbContextOptionsBuilder optionsBuilder)
// {
// if (!optionsBuilder.IsConfigured)
// {
//#warning To protect potentially sensitive information
in your connection string, you should move it out of
source code. See http://go.microsoft.com/fwlink/?LinkId=723263
for guidance on storing connection strings.
// optionsBuilder.UseSqlServer(@"Server=.;
Database=FlixOneStore;Trusted_Connection=True;");
// }
// }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Customers>(entity =>
{
entity.Property(e => e.Id)
.HasColumnName("id")
.ValueGeneratedNever();
entity.Property(e => e.Dob)
.HasColumnName("dob")
.HasColumnType("datetime");
entity.Property(e => e.Email)
.IsRequired()
.HasColumnName("email")
.HasMaxLength(110);
entity.Property(e => e.Fax)
.IsRequired()
.HasColumnName("fax")
.HasMaxLength(50);
entity.Property(e => e.Firstname)
.IsRequired()
.HasColumnName("firstname")
.HasMaxLength(50);
entity.Property(e => e.Gender)
.IsRequired()
.HasColumnName("gender")
.HasColumnType("char(1)");
entity.Property(e => e.Lastname)
.IsRequired()
.HasColumnName("lastname")
.HasMaxLength(50);
entity.Property(e => e.Mainaddressid).HasColumnName
("mainaddressid");
entity.Property(e => e.Newsletteropted).HasColumnName
("newsletteropted");
entity.Property(e => e.Password)
.IsRequired()
.HasColumnName("password")
.HasMaxLength(50);
entity.Property(e => e.Telephone)
.IsRequired()
.HasColumnName("telephone")
.HasMaxLength(50);
});
}
}
}
Did you notice that I have commented the OnConfiguring method and added a constructor so that we can inject dependencies from startup to initialize the context with a connection string? Let's do that.
So, inside the ConfigureServices startup, we will add the context to the services collection using the connection string:
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IProductService, ProductService>();
services.AddMvc();
var connection = @"Server=.;Database=FlixOneStore;
Trusted_Connection=True";
services.AddDbContext<FlixOneStoreContext>(
options => options.UseSqlServer(connection));
}