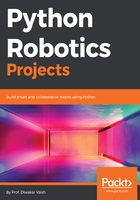
A deeper pe into GPIOs
I am sure you remember this line of code from the previous chapter:
GPIO.setup(18,GPIO.OUT)
As explained earlier, this basically tells us how GPIO the pin will behave in a certain program. By now, you must have guessed that by changing this single line of code we can change the behavior of the pin and convert it from output to input. This is how you would do it:
GPIO.setup(18,GPIO.IN)
Once you write this line of code in your program, the microcontroller will know that during the time that the program is being run, the pin number 18 will only be used for input purposes.
To understand how this would actually work, let's head back to our hardware and see how it can be done. Firstly, you need to connect an LED to any of the pins; we will be using pin number 23 in this program. Secondly, you need to connect a switch on pin number 24. You can refer the diagram that follows for making the connections:

Once you connect it, you can go ahead and write this program:
import time import RPi.GPIO as GPIO
GPIO.setmode(GPIO.BCM)
GPIO.setup(24,GPIO.IN)
GPIO.setup(23,GPIO.OUT)
while True:
button_state = GPIO.input(24)
if button_state == True:
GPIO.output(23,GPIO.HIGH)
else:
GPIO.output(23,GPIO.LOW)
time.sleep(0.5)
GPIO.cleanup()
Once the program is uploaded, then, as soon as you press the push button, the LED will turn itself on.
Let's understand what exactly is happening. while True: is basically an infinite loop; once you apply this loop, the code running inside it is repeated over and over again until something breaks it, and by break I mean some interruption that causes the program to stop and exit. Now, ideally we exit the program by pressing Ctrl + C whenever there is an infinite loop.
button_state = GPIO.input(24)
In the above line, the program understands where it has to look; in this program. In this line we are telling the program that we are looking for GPIO 24, which is an input:
if button_state == True:
GPIO.output(23,GPIO.HIGH)
If the button is high, in other words when the button is pressed and the current is reaching the pin number 24, then the GPIO pin number 23 will be set to high:
else:
GPIO.output(23,GPIO.LOW)
If the pin number 24 is not true, it will follow this line of code and will keep the pin number 23 low, in other words switched off.
So, there it is, your first program for using the GPIOs for input purposes.