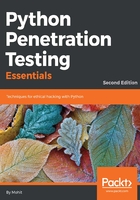
Socket exceptions
In order to handle exceptions, we'll use the try and except blocks. The following example will tell you how to handle the exceptions. Run udptime2.py:
import socket host = "192.168.0.1" port = 12346 s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) try: s.bind((host,port)) s.settimeout(5) data, addr = s.recvfrom(1024) print "recevied from ",addr print "obtained ", data s.close() except socket.timeout : print "Client not connected" s.close()
The output is shown in the following screenshot:

In the try block, I put my code, and from the except block, a customized message is printed if any exception occurs.
Different types of exceptions are defined in Python's socket library for different errors. These exceptions are described here:
- exception socket.herror: This block catches the address-related error.
- exception socket.timeout: This block catches the exception when a timeout on a socket occurs, which has been enabled by settimeout(). In the previous example, you can see that we used socket.timeout.
- exception socket.gaierror: This block catches any exception that is raised due to getaddrinfo() and getnameinfo().
- exception socket.error: This block catches any socket-related errors. If you are not sure about any exception, you could use this. In other words, you can say that it is a generic block and can catch any type of exception.
Downloading the example code
You can download the example code files from your account at http://www.packtpub.com for all of the Packt Publishing books you have purchased. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files emailed directly to you.