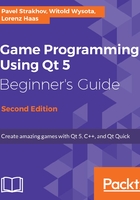
Properties
In Chapter 3, Qt GUI Programming, we edited predefined properties of widgets in the form editor and used their getter and setter methods in the code. However, until now, there wasn't a real reason for us to declare a new property. It'll be useful with the Animation framework, so let's pay more attention to properties.
Only classes that inherit QObject can declare properties. To create a property, we first need to declare it in a private section of the class (usually right after the Q_OBJECT mandatory macro) using a special Q_PROPERTY macro. That macro allows you to specify the following information about the new property:
- The property name—a string that identifies the property in the Qt meta system.
- The property type—any valid C++ type can be used for a property, but animations will only work with a limited set of types.
- Names of the getter and setter method for this property. If declared in Q_PROPERTY, you must add them to your class and implement them properly.
- Name of the signal that is emitted when the property changes. If declared in Q_PROPERTY, you must add the signal and ensure that it's properly emitted.
There are more configuration options, but they are less frequently needed. You can learn more about them from the The Property System documentation page.
Similar to signals and slots, properties are powered by moc, which reads the header file of your class and generates extra code that enables Qt (and you) to access the property at runtime. For example, you can use the QObject::property() and QObject::setProperty() methods to get and set properties by name.