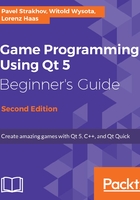
Have a go hero - Letting the item handle Benjamin's jump
Since the scene is already a QObject, adding a property to it was easy. However, imagine that you want to create a game for two players, each controlling a separate Player item. In this case, the jump factors of two elephants need to be animated independently, so you want to make an animated property in the Player class, instead of putting it to the scene.
The QGraphicsItem item and all standard items introduced so far don't inherit QObject and thus can't have slots or emit signals; they don't benefit from the QObject property system either. However, we can make them use QObject! All you have to do is add QObject as a base class and add the Q_OBJECT macro:
class Player : public QObject, public QGraphicsPixmapItem { Q_OBJECT
//...
};
Now you can use properties, signals, and slots with items too. Be aware that QObject must be the first base class of an item.
Only use QObject with items if you really need its capabilities. QObject
adds a lot of overhead to the item, which will have a noticeable impact on performance when you have many items, so use it wisely and not only because you can.
If you make this change, you can move the jumpFactor property from MyScene to Player, along with a lot of related code. You can make the code even more consistent by handling the horizontal movement in Player as well. Let MyScene handle the input events and forward the movement commands to Player.