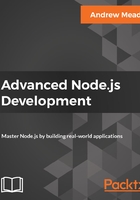
The deleteOne method
The goal here is to use deleteOne to delete the document where the text equals Eat lunch, but since we're using deleteOne and not deleteMany, one of these should stay around and one of them should go away.
Back inside of Atom, we can go ahead and get started by calling db.collection with the collection name we want to target. In this case it's Todos again, and we're going to use deleteOne. The deleteOne method takes that same criteria. We're going to target documents where text equals Eat lunch.
This time though, instead of deleting multiple documents we're just going to delete the one, and we are still going to get that same exact result. To prove it, I'll just print to the screen like we did previously with console.log(result):
//deleteOne db.collection('Todos').deleteOne({text: 'Eat lunch'}).then((result) => { console.log(result); });
With this in place, we can now rerun our script and see what happens. In the Terminal, I'm going to shut down our current connection and rerun it:

We get a similar-looking object, a bunch of junk we don't really care about, but once again if we scroll to the top we have a result object, where ok is 1 and the number of deleted documents is also 1. Even though multiple documents did pass this criteria it only deleted the first one, and we can prove that by going over to Robomongo, right-clicking up above, and viewing the documents again. This time around, we have three Todos.
We do still have one of the Todos with the Eat lunch text:

And now that we know how to use these two methods, I want to take a look at my favorite method. This is findOneAndDelete.