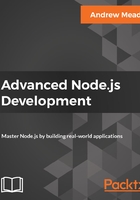
Getting body data from the client
To do this, we have to use the body-parser module. As I mentioned previously, body-parser is going to take your JSON and convert it into an object, attaching it onto this request object. We're going to configure the middleware using app.use. The app.use takes the middleware. If we're writing custom middleware, it'll be a function; if we're using third-party middleware, we usually just access something off of the library. In this case, it's going to be bodyParser.json getting called as a function. The return value from this JSON method is a function, and that is the middleware that we need to give to Express:
var app = express();
app.use(bodyParser.json());
With this in place, we can now send JSON to our Express application. What I'd like to do inside of the post callback is simply console.log the value of req.body, where the body gets stored by bodyParser:
app.use(bodyParser.json());
app.post('/todos', (req, res) => {
console.log(req.body);
});
We can now start up the server and test things out inside of Postman.
Testing the POST route inside Postman
In the Terminal, I'm going to use clear to clear the Terminal output, and then I'll run the app:
node server/server.js
The server is up on port 3000, which means we can now head into Postman:

Inside of Postman, we're not going to be making a GET request like we did in the previous section. This time, what we're going to be doing is making a POST request, which means we need to change the HTTP method to POST, and type the URL. That's going to be localhost:3000 for the port, /todos. This is the URL that we want to send our data to:

Now in order to send some data to the application, we have to go to the Body tab. We're trying to send JSON data, so we're going to go to raw and select JSON (application/json) from the drop-down list on the right-hand side:

Now we have our Header set. This is the Content-Type header, letting the server know that JSON is getting sent. All of this is done automatically with Postman. Inside of Body, the only piece of information I'm going to attach to my JSON is a text property:
{ "text": "This is from postman" }
Now we can click Send to fire off our request. We're never going to get a response because we haven't responded to it inside of server.js, but if I head over to the Terminal, you see we have our data:

This is the data we created inside of Postman. It's now showing up in our Node application, which is fantastic. We are one step closer to actually creating that Todo. The only thing left to do inside of the post handler is to actually create the Todo using the information that comes from the User.