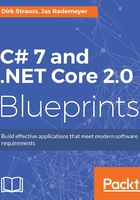
Throw expressions
We also need to add the code to save a new storage space. Add the following code to the Click event of the btnSaveNewStorageSpace button:
private void btnSaveNewStorageSpace_Click(object sender,
EventArgs e) { try { if (txtNewStorageSpaceName.Text.Length != 0) { string newName = txtNewStorageSpaceName.Text; // throw expressions: bool spaceExists =
(space exists = false) ? return false : throw exception // Out variables bool spaceExists = (!spaces.StorageSpaceExists
(newName, out int nextID)) ? false : throw new
Exception("The storage space you are
trying to add already exists."); if (!spaceExists) { StorageSpace newSpace = new StorageSpace(); newSpace.Name = newName; newSpace.ID = nextID; newSpace.Description =
txtStorageSpaceDescription.Text; spaces.Add(newSpace); PopulateStorageSpacesList(); // Save new Storage Space Name txtNewStorageSpaceName.Clear(); txtNewStorageSpaceName.Visible = false; lblStorageSpaceDescription.Visible = false; txtStorageSpaceDescription.ReadOnly = true; txtStorageSpaceDescription.Clear(); btnSaveNewStorageSpace.Visible = false; btnCancelNewStorageSpaceSave.Visible = false; dlVirtualStorageSpaces.Enabled = true; btnAddNewStorageSpace.Enabled = true; } } } catch (Exception ex) { txtNewStorageSpaceName.SelectAll(); MessageBox.Show(ex.Message); } }
Here, we can see another new feature in the C# 7 language called throw expressions. This gives developers the ability to throw exceptions from expressions. The code in question is this code:
bool spaceExists = (!spaces.StorageSpaceExists(newName, out int nextID)) ? false : throw new Exception("The storage space you are trying to add already exists.");
I always like to remember the structure of the code as follows:

The last few methods deal with saving eBooks in the selected Virtual Storage Space. Modify the Click event of the btnAddBookToStorageSpace button. This code also contains a throw expression. If you haven't selected a storage space from the combo box, a new exception is thrown:
private void btnAddeBookToStorageSpace_Click(object sender, EventArgs e) { try { int selectedStorageSpaceID =
dlVirtualStorageSpaces.SelectedValue.ToString().ToInt(); if ((selectedStorageSpaceID !=
(int)StorageSpaceSelection.NoSelection)
&& (selectedStorageSpaceID !=
(int)StorageSpaceSelection.New)) { UpdateStorageSpaceBooks(selectedStorageSpaceID); } else throw new Exception("Please select a Storage
Space to add your eBook to"); // throw expressions } catch (Exception ex) { MessageBox.Show(ex.Message); } }
Developers can now immediately throw exceptions in code right there where they occur. This is rather nice and makes code cleaner and its intent clearer.