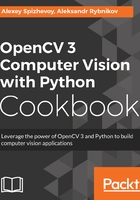
上QQ阅读APP看书,第一时间看更新
How to do it...
To get the result, it's necessary to go through a few steps:
- Import all necessary modules:
import cv2, numpy as np
- Create a matrix of a certain shape and fill it with 255 as a value, which should display the following:
image = np.full((480, 640, 3), 255, np.uint8)
cv2.imshow('white', image)
cv2.waitKey()
cv2.destroyAllWindows()
- Create a matrix and set individual values for the colors of each pixel to color our matrix red:
image = np.full((480, 640, 3), (0, 0, 255), np.uint8)
cv2.imshow('red', image)
cv2.waitKey()
cv2.destroyAllWindows()
- Fill our matrix with zeros to make it black:
image.fill(0)
cv2.imshow('black', image)
cv2.waitKey()
cv2.destroyAllWindows()
- Next, set some individual pixels' values to white:
image[240, 160] = image[240, 320] = image[240, 480] = (255, 255, 255)
cv2.imshow('black with white pixels', image)
cv2.waitKey()
cv2.destroyAllWindows()
- Now, let's set the first channel of all pixels to 255 to make black ones blue:
image[:, :, 0] = 255
cv2.imshow('blue with white pixels', image)
cv2.waitKey()
cv2.destroyAllWindows()
- Now, set pixels on a vertical line in the middle of the image to white:
image[:, 320, :] = 255
cv2.imshow('blue with white line', image)
cv2.waitKey()
cv2.destroyAllWindows()
- Finally, set the second channel of all pixels inside a certain region to 255:
image[100:600, 100:200, 2] = 255
cv2.imshow('image', image)
cv2.waitKey()
cv2.destroyAllWindows()