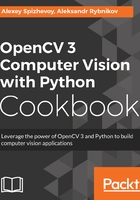
上QQ阅读APP看书,第一时间看更新
How to do it...
The following steps are required for this recipe:
- Import all necessary modules, open an image, print its shape and data type, and display it on the screen:
import cv2, numpy as np
image = cv2.imread('../data/Lena.png')
print('Shape:', image.shape)
print('Data type:', image.dtype)
cv2.imshow('image', image)
cv2.waitKey()
cv2.destroyAllWindows()
- Convert our image to one with floating data type elements:
image = image.astype(np.float32) / 255
print('Shape:', image.shape)
print('Data type:', image.dtype)
- Scale the elements of our image by 2 and clip the values to keep them in the [0, 1] range:
cv2.imshow('image', np.clip(image*2, 0, 1))
cv2.waitKey()
cv2.destroyAllWindows()
- Scale the elements of our image back to the [0, 255] range, and convert the element type to 8-bit unsigned int:
image = (image * 255).astype(np.uint8)
print('Shape:', image.shape)
print('Data type:', image.dtype)
cv2.imshow('image', image)
cv2.waitKey()
cv2.destroyAllWindows()