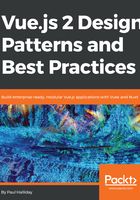
Mobile development
When it comes to developing mobile applications, projects such as Angular and React are great choices for developing mobile-first applications. The success of the NativeScript, React Native, and Ionic Framework projects have boosted the significant popularity of these frameworks. For instance, Ionic Framework currently has more stars than Angular on GitHub!
Vue is making waves in this area with projects such as NativeScript Vue, Weex, and Quasar Framework. All of the listed projects are relatively new, but it only takes one to truly spike the popularity of Vue in production even further. Using NativeScript Vue as an example, it only takes 43 lines of code to create a cross-platform mobile application that connects to a REST API and displays the results on screen. If you'd like to follow along with this yourself, run:
# Install the NativeScript CLI npm install nativescript -g # New NativeScript Vue project tns create NSVue --template nativescript-vue-template # Change directory cd NSVue # Run on iOS tns run ios
Then, we can place the following inside of our app/app.js:
const Vue = require('nativescript-vue/dist/index'); const http = require('http'); Vue.prototype.$http = http; new Vue({ template: ` <page>
<action-bar class="action-bar" title="Posts"></action-bar>
<stack-layout>
<list-view :items="posts">
<template scope="post">
<stack-layout class="list">
<label :text="post.title"></label>
<label :text="post.body"></label>
</stack-layout>
</template>
</list-view>
</stack-layout>
</page>
`, data: { posts: [] }, created(args) { this.getPosts(); }, methods: { getPosts() { this.$http .getJSON(`https://jsonplaceholder.typicode.com/posts`) .then(response => { this.posts = response.map( post => { return { title: post.title, body: post.body } } ) }); } } }).$start();
If we then run our code, we can see a list of posts. You'll notice that our Vue code is declarative, and we have the power of larger frameworks at our disposal with much less code:
