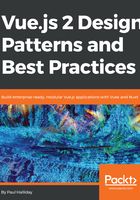
JavaScript modules
In order to create reusable modular code, our aim should be to have one file per feature in most cases. This allows us to avoid the dreaded "Spaghetti code" anti-pattern, where we have strong coupling and little separation of concerns. Continuing with the pasta-oriented theme, the solution to this is to embrace the "Ravioli code" pattern with smaller, loosely coupled, distributed modules that are easier to work with. What does a JavaScript module look like?
In ECMAScript2015, a module is simply a file that uses the export keyword, and allows other modules to then import that piece of functionality:
// my-module.js
export default function add(x, y) {
return x + y
}
We could then import add from another module:
// my-other-module.js
import { add } from './my-other-module'
add(1, 2) // 3
As browsers haven't fully caught up with module imports yet, we often use tools to assist with the bundling process. Common projects in this area are Babel, Bublé, Webpack, and Browserify. When we create a new project with the Webpack template, it uses the Vue-loader to transform our Vue components into a standard JavaScript module.