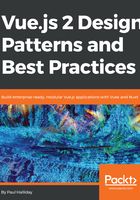
Lifecycle hooks
Lifecycle hooks such as created(), mounted(), destroyed(), and so on can be defined as functions within the class.
- created()
This allows for actions to be performed to a component before it is added into the DOM. Using this hook allows access to both data and events.
- mounted()
Mounted gives access to a component before it is rendered as well as after it has been rendered. It provides full access for interacting with the DOM and component.
- destroyed()
Everything that was attached to the component has been destroyed. It allows for cleanup of the component when it is removed from the DOM.
They'll be recognized and act the same way as expected without TypeScript. Here's an example when using the created and mounted hooks:
// Omitted
export default class App extends Vue {
name: string = 'Paul';
created() {
console.log(`Created: Hello ${this.name}`)
}
mounted() {
console.log(`Mounted: Hello ${this.name}`);
}
}
Now if we head over to the console, we can see that the message of 'Hello' is outputted with the name of Paul:
