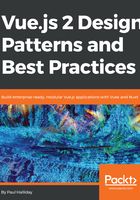
上QQ阅读APP看书,第一时间看更新
Computed
Computed properties allow for multiple expressions to be passed, and these properties on the Vue instance require the use of class getters/setters. So, if we wanted to retrieve a reversed version of our name, we could simply pass the following:
@Component({
template: `
<div>
<input type="text" v-model="name" />
<button @click="sayHello(name)">Say Hello!</button>
<p>{{nameReversed}}</p>
</div>
`
})
export default class App extends Vue {
@Prop({ default: 'Paul Halliday' }) name: string;
// Computed values
get nameReversed() {
return this.name.split("").reverse().join("");
}
sayHello(name: string): void {
alert(`Hello ${name}`)
}
}
This would otherwise have been equivalent to:
export default {
computed: {
nameReversed() {
return this.name.split("").reverse().join("");
}
}
}
Other decorators can be used, such as @Watch, @Inject, @Model, and @Provide. Each decorator allows for a consistent implementation, and provides a similar API to the vanilla Vue instance. In the next section, we're going to look at how we can enhance the reactivity of our Vue applications with RxJS.