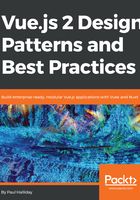
How Vue handles 'this'
You may have noticed up to this point that we're able to reference values inside of our data, methods, and other objects using this syntax, but the actual structure of our instance is this.data.propertyName or this.methods.methodName; all of this is possible due to the way Vue proxies our instance properties.
Let's take a very simple Vue application that has one instance. We have a data object that has a message variable and a method named showAlert; how does Vue know how to access our alert text with this.message?
<template>
<button @click="showAlert">
Show Alert</button>
</template>
<script>
export default {
data() {
return {
message: 'Hello World!',
};
},
methods: {
showAlert() {
alert(this.message);
},
},
};
</script>
Vue proxies the instance properties to the top level object, allowing us to access these properties via this. If we were to log out the instance to the console (with the help of Vue.js devtools), we'd get the following result:

The key properties to look at within the preceding screenshot are message and showAlert, both of which are defined on our Vue instance yet proxied to the root object instance at initialization time.