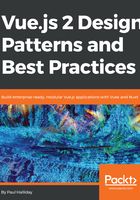
Lifecycle hooks
We have access to a variety of lifecycle hooks that fire at particular points during the creation of our Vue instance. These hooks range from prior to creation with beforeCreate, to after the instance is mounted, destroyed, and many more in between.
As the following figure shows, the creation of a new Vue instance fires off functions at varying stages of the instance lifecycle.
We'll be looking at how we can activate these hooks within this section:

Taking advantage of the lifecycle hooks (https://vuejs.org/v2/guide/instance.html) can be done in a similar way to any other property on our Vue instance. Let's take a look at how we can interact with each one of the hooks, starting from the top; I'll be creating another project based on the standard webpack-simple template:
// App.vue
<template>
</template>
<script>
export default {
data () {
return {
msg: 'Welcome to Your Vue.js App'
}
},
beforeCreate() {
console.log('beforeCreate');
},
created() {
console.log('created');
}
}
</script>
Notice how we've simply added these functions to our instance without any extra imports or syntax. We then get two different log statements in our console, one prior to the creation of our instance and one after it has been created. The next stage for our instance is the beforeMounted and mounted hooks; if we add these, we'll be able to see a message on the console once again:
beforeMount() {
console.log('beforeMount');
},
mounted() {
console.log('mounted');
}
If we then modified our template so it had a button that updated one of our data properties, we'd be able to fire a beforeUpdated and updated hook:
<template>
<div>
<h1>{{msg}}</h1>
<button @click="msg = 'Updated Hook'">Update Message</button>
</div>
</template>
<script>
export default {
data () {
return {
msg: 'Welcome to Your Vue.js App'
}
},
beforeCreate() {
console.log('beforeCreate');
},
created() {
console.log('created');
},
beforeMount() {
console.log('beforeMount');
},
mounted() {
console.log('mounted');
},
beforeUpdated() {
console.log('beforeUpdated');
},
updated() {
console.log('updated');
}
}
</script>
Whenever we select the Update Message button, our beforeUpdated and updated hooks both fire. This allows us to perform an action at this stage in the lifecycle, leaving us only with beforeDestroy and destroyed yet to cover. Let's add a button and a method to our instance that call $destroy, allowing us to trigger the appropriate lifecycle hook:
<template>
<div>
<h1>{{msg}}</h1>
<button @click="msg = 'Updated Hook'">Update Message
</button>
<button @click="remove">Destroy instance</button>
</div>
</template>
We can then add the remove method to our instance, as well as the functions that allow us to capture the appropriate hooks:
methods: {
remove(){
this.$destroy();
}
},
// Other hooks
beforeDestroy(){
console.log("Before destroy");
},
destroyed(){
console.log("Destroyed");
}
When we select the destroy instance button, the beforeDestroy and destroy lifecycle hooks will fire. This allows us to clean up any subscriptions or perform any other action(s) when destroying an instance. In a real-world scenario, lifecycle control should be left up to data-driven directives, such as v-if and v-for. We'll be looking at these directives in more detail in the next chapter.