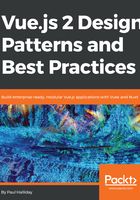
DOM
The DOM is what is used to describe the structure of an HTML or XML page. It creates a tree-like structure that provides us with the ability to do everything from creating, reading, updating, and deleting nodes to traversing the tree and many more features, all within JavaScript. Let's consider the following HTML page:
<!DOCTYPE html>
<html lang="en">
<head>
<title>DOM Example</title>
</head>
<body>
<div>
<p>I love JavaScript!</p>
<p>Here's a list of my favourite frameworks:</p>
<ul>
<li>Vue.js</li>
<li>Angular</li>
<li>React</li>
</ul>
</div>
<script src="app.js"></script>
</body>
</html>
We're able to look at the HTML and see that we have one div, two p, one ul, and li tags. The browser parses this HTML and produces the DOM Tree, which at a high level looks similar to this:

We can then interact with the DOM to get access to these elements by TagName using document.getElementsByTagName(), returning a HTML collection. If we wanted to map over these collection objects, we could create an array of these elements using Array.from. The following is an example:
const paragraphs = Array.from(document.getElementsByTagName('p'));
const listItems = Array.from(document.getElementsByTagName('li'));
paragraphs.map(p => console.log(p.innerHTML));
listItems.map(li => console.log(li.innerHTML));
This should then log the innerHTML of each item to the console inside of our array(s), thus showing how we can access items inside of the DOM:
