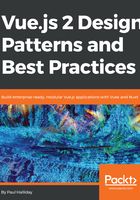
How 'this' works within JavaScript
Within JavaScript, this has varying contexts that range from the global window context to eval, newable, and function contexts. As the default context for this relates to the global scope, this is our window object:
/**
* Outputting the value of this to the console in the global context returns the Window object
*/
console.log(this);
/**
* When referencing global Window objects, we don't need to refer to them with this, but if we do, we get the same behavior
*/
alert('Alert one');
this.alert('Alert two');
The context of this changes depending on where we are in scope. This means, that if we had a Student object with particular values, such as firstName, lastName, grades, and so on, the context of this would be related to the object itself:
/**
* The context of this changes when we enter another lexical scope, take our Student object example:
*/
const Student = {
firstName: 'Paul',
lastName: 'Halliday',
grades: [50, 95, 70, 65, 35],
getFullName() {
return `${this.firstName} ${this.lastName}`
},
getGrades() {
return this.grades.reduce((accumulator, grade) => accumulator + grade);
},
toString() {
return `Student ${this.getFullName()} scored ${this.getGrades()}/500`;
}
}
When we run the preceding code with console.log(Student.toString()), we get this: Student Paul Halliday scored 315/500 as the context of this is now scoped to the object itself rather than the global window scope.
If we wanted to display this in the document we could do it like so:
let res = document.createTextNode(Student.toString());
let heading = document.createElement('h1');
heading.appendChild(res);
document.body.appendChild(heading);
Notice that, with the preceding code, once again we don't have to use this as it isn't needed with the global context.
Now that we have an understanding of how this works at a basic level, we can investigate how Vue proxies this inside of our instances to make interacting with the various properties much easier.