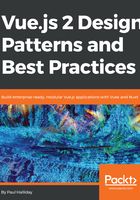
Data properties
When we add a variable to our data object, we're essentially creating a reactive property that updates the view any time it changes. This means that, if we had a data object with a property named firstName, that property would be re-rendered on the screen each time the value changes:
<!DOCTYPE html>
<html>
<head>
<title>Vue Data</title>
<script src="https://unpkg.com/vue"></script>
</head>
<body>
<div id="app">
<h1>Name: {{ firstName }}</h1>
<input type="text" v-model="firstName">
</div>
<script>
const app = new Vue({
el: '#app',
data: {
firstName: 'Paul'
}
});
</script>
</body>
</html>
This reactivity does not extend to objects added to our Vue instance after the instance has been created outside of the data object. If we had another example of this, but this time including appending another property such as fullName to the instance itself:
<body>
<div id="app">
<h1>Name: {{ firstName }}</h1>
<h1>Name: {{ name }}</h1>
<input type="text" v-model="firstName">
</div>
<script>
const app = new Vue({
el: '#app',
data: {
firstName: 'Paul'
}
});
app.fullName = 'Paul Halliday';
</script>
</body>
Even though this item is on the root instance (the same as our firstName variable), fullName is not reactive and will not re-render upon any changes. This does not work because, when the Vue instance is initialized, it maps over each one of the properties and adds a getter and setter to each data property, thus, if we add a new property after initialization, it lacks this and is not reactive.
How does Vue achieve reactive properties? Currently, it uses Object.defineProperty to define a custom getter/setter for items inside of the instance. Let's create our own property on an object with standard get/set features:
const user = {};
let fullName = 'Paul Halliday';
Object.defineProperty(user, 'fullName', {
configurable: true,
enumerable: true,
get() {
return fullName;
},
set(v) {
fullName = v;
}
});
console.log(user.fullName); // > Paul Halliday
user.fullName = "John Doe";
console.log(user.fullName); // > John Doe
As the watchers are set with a custom property setter/getter, merely adding a property to the instance after initialization doesn't allow for reactivity. This is likely to change within Vue 3 as it will be using the newer ES2015+ Proxy API (but potentially lacking support for older browsers).
There's more to our Vue instance than a data property! Let's use computed to create reactive, derived values based on items inside of our data model.