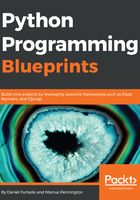
Creating the Flask application
Perfect, now let's add another file called twitter_auth.py in the twittervotes directory. We are going to create three functions in it but, first, let's add some imports:
from urllib.parse import parse_qsl
import yaml
from flask import Flask
from flask import render_template
from flask import request
import oauth2 as oauth
from core import read_config
from core.models import RequestToken
First, we import the parser_qls from the urllib.parse module to parse the returned query string, and the yaml module so we can read and write YAML configuration files. Then we import everything we need to build our Flask application. The last third-party module that we are going to import here is the oauth2 module, which will help us to perform the OAuth authentication.
Lastly, we import our function read_config and the RequestToken namedtuple that we just created.
Here, we create our Flask app and a few global variables that will hold values for the client, consumer, and the RequestToken instance:
app = Flask(__name__)
client = None
consumer = None
req_token = None
The first function that we are going to create is a function called get_req_token with the following content:
def get_oauth_token(config):
global consumer
global client
global req_token
consumer = oauth.Consumer(config.consumer_key,
config.consumer_secret)
client = oauth.Client(consumer)
resp, content = client.request(config.request_token_url, 'GET')
if resp['status'] != '200':
raise Exception("Invalid response
{}".format(resp['status']))
request_token = dict(parse_qsl(content.decode('utf-8')))
req_token = RequestToken(**request_token)
This function gets as argument an instance to the configuration and the global statements say to the interpreter that the consumer, client, and req_token used in the function will be referencing the global variables.
We create a consumer object using the consumer key and the consumer secret that we obtained when the Twitter app was created. When the consumer is created, we can pass it to the client function to create the client, then we call the function request, which, as the name suggests, will perform the request to Twitter, passing the request token URL.
When the request is complete, the response and the content will be stored in the variables resp and content. Right after that, we test whether the response status is not 200 or HTTP.OK; in that case, we raise an exception, otherwise we parse the query string to get the values that have been sent back to us and create a RequestToken instance.