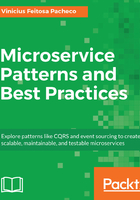
Python
In the world of Python, there is a multitude of interesting frameworks; Bottle, Pyramid, Flask, Sanic, JaPronto, Tornado, and Twisted are some examples. The most famous that has more support from the community is Django.
Django is the same framework that was used to build our news portal in the monolithic version. It is a very good tool for full-stack applications and has, the main characteristic, and the simplicity to mount this type of software.
However, for microservices, I do not think it's the best framework for this purpose. Firstly, because the exposure of APIs is not native to Django. The ability to create an API with Django comes from a Django app called Django Framework Rest. In order to maintain the standard structure of the Django framework, the design of a simple API was a bit compromised. Django provides the developer with the entire development stack, which is not always what you want when developing microservices. A more simplistic and more flexible framework for compositions can be interesting.
In the Python ecosystem, there are other frameworks that work very well when it comes to APIs. Flask is a good example. An API, Hello world, is made in a very simple way.
Installing Flask with the command:
$ pip install flask
Writing the endpoint is very simple:
# file: app.py # import de dependencies from flask import Flask, jsonify
Instantiating the framework:
app = Flask(__name__)
Declaring the route:
@app.route('/') def hello(): #Prepare the json to return return jsonify({'hello': 'world'})
Executing the main app:
if __name__ == '__main__': app.run()
The preceding code is enough to return the message hello world in JSON format.
Other frameworks were inspired by Flask and worship the same simplicity and very similar syntax. A good example is Sanic.
Sanic can be installed with the command:
$ pip install sanic
The syntax is almost identical to Flask, as can be seen in the following code:
# file: app.py
Importing the dependencies:
from sanic import Sanic from sanic.response import json
Instantiating the framework:
app = Sanic()
Declaring the route:
@app.route("/") async def test(request): #Prepare the json to return return json({"hello": "world"})
Executing the main app:
if __name__ == "__main__": app.run(host="0.0.0.0", port=8000)
The big difference between Flask and Sanic is the performance. The performance of Sanic is much higher than Flask due to use of newer features in Python and the possibility of the exchange of the Sanic framework. However, Sanic is still not as mature and experienced in the market as Flask.
Another plus point for Flask is the integration with other tools like Swagger. Using the two together, our APIs would be not only written but also documented appropriately.
For microservices using Python as a programming language, performance is not the most important requirement, but practicality. Use this framework as Flask microservices.