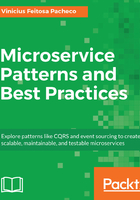
RabbitMQ
RabbitMQ uses AMQP, by default, as the communication protocol, which makes this a very performative tool for the delivery of messages. RabbitMQ documentation is incredible and has native support for the languages most used in the market programming.
Among the many message brokers, RabbitMQ stands out because of its practicality of implementation, flexibility to be coupled with other tools, and its simple and intuitive API.
The following code shows how simple it is to create a system of Hello World in Python with RabbitMQ:
# import the tool communicate with RabbitMQ import pika # create the connection connection = pika.BlockingConnection( pika.ConnectionParameters(host='localhost')) # get a channel from RabbitMQ channel = connection.channel() # declare the queue channel.queue_declare(queue='hello') # publish the message channel.basic_publish(exchange='', routing_key='hello', body='Hello World!') print(" [x] Sent 'Hello World!'") # close the connection connection.close()
With the preceding example, we took the official RabbitMQ site, we are responsible for sending the message Hello World queue for the hello. The following is the code that gets the message:
# import the tool communicate with RabbitMQ import pika # create the connection connection = pika.BlockingConnection( pika.ConnectionParameters(host='localhost')) # get a channel from RabbitMQ channel = connection.channel() # declare the queue channel.queue_declare(queue='hello') # create a method where we'll work with the message received def callback(ch, method, properties, body): print(" [x] Received %r" % body) # consume the message from the queue passing to the method
created above channel.basic_consume(callback, queue='hello', no_ack=True) print(' [*] Waiting for messages. To exit press CTRL+C') # keep alive the consumer until interrupt the process channel.start_consuming()
The code is very simple and readable. Another feature of RabbitMQ is the practical tool for scalability. There are performance tests that indicate the ability of RabbitMQ in supporting 20,000 messages per second for each node.